Java
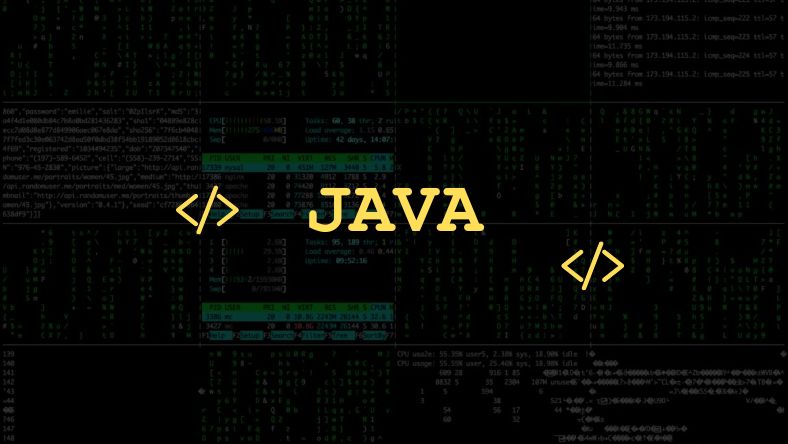
Contact Form
Java is a versatile, object-oriented programming language used for building a wide range of applications, from web to mobile and enterprise systems. Known for its “write once, run anywhere” capability, Java operates across platforms and devices. With robust libraries, frameworks, and a strong developer community, it enables scalable, secure, and efficient software solutions in diverse global industries.
- Basic knowledge of computer programming concepts will be beneficial.
- Understanding of object-oriented programming principles and familiarity with basic computer operations and networking will enhance the learning experience in Java.
- Previous experience with any programming language is helpful but not required.
Module 1: Introduction to Java
Overview of Java
- History of Java and its evolution.
- Characteristics of Java: Platform independence, Object-Oriented, and Robust.
- Java Development Kit (JDK), Java Runtime Environment (JRE), and Java Virtual Machine (JVM).
Setting up Java Development Environment
- Installing JDK and setting up IDE (Eclipse, IntelliJ IDEA, NetBeans).
- Writing and executing the first Java program.
Understanding Java Syntax
- Structure of a Java program (classes, methods, main function).
- Basic syntax rules: keywords, identifiers, operators.
- Comments, Data types, and Variables.
Module 2: Java Basics
Primitive Data Types
- Integer types:
byte
,short
,int
,long
. - Floating-point types:
float
,double
. - Other types:
char
,boolean
.
- Integer types:
Control Flow Statements
- Conditional statements:
if
,else
,switch
. - Looping statements:
for
,while
,do-while
.
- Conditional statements:
Arrays
- Declaring and initializing arrays.
- Multidimensional arrays.
- Array manipulation (sorting, searching).
Methods and Functions
- Defining and invoking methods.
- Method parameters and return types.
- Method overloading.
Module 3: Object-Oriented Programming (OOP) Concepts
Introduction to OOP
- What is OOP? The four pillars of OOP: Encapsulation, Abstraction, Inheritance, Polymorphism.
Classes and Objects
- Defining classes and creating objects.
- Constructor methods and their role in object creation.
Encapsulation
- Understanding access modifiers:
private
,public
,protected
, anddefault
. - Getter and setter methods.
- Understanding access modifiers:
Inheritance
- Extending classes and inheriting properties.
- Method overriding and
super
keyword.
Polymorphism
- Method overloading and method overriding.
- Compile-time vs Runtime polymorphism.
Abstraction
- Abstract classes and interfaces.
- Abstract methods and their use cases.
Module 4: Java Advanced Concepts
Exception Handling
- Try-catch blocks.
- Throwing and catching exceptions.
- Creating custom exceptions.
Collections Framework
- Introduction to Collections: List, Set, Map.
- ArrayList, HashSet, HashMap, TreeMap.
- Iterating through collections.
- Understanding
Iterator
interface.
Generics
- Introduction to generics and why they are useful.
- Generic classes and methods.
- Type bounds and wildcards.
Java I/O
- File handling: Reading and writing files.
- Streams: InputStream, OutputStream, Reader, Writer.
- FileReader, FileWriter, BufferedReader, BufferedWriter.
Module 5: Java Multithreading
Introduction to Multithreading
- What is multithreading and why is it important?
- Creating threads using
Thread
class andRunnable
interface.
Thread Lifecycle and Synchronization
- Thread states: New, Runnable, Blocked, Waiting, Terminated.
- Thread synchronization and preventing race conditions.
- Using
synchronized
keyword andLock
interface.
Concurrency Utilities
- Executor framework.
- Callable and Future.
- Thread pooling.
Module 6: Java Memory Management
- Understanding Java Memory Model
- Stack and Heap memory.
- Understanding the role of the Garbage Collector.
- Automatic Garbage Collection
- How garbage collection works in Java.
- Manual garbage collection with
System.gc()
.
- Memory Leaks and Optimization
- Identifying and avoiding memory leaks.
- Optimizing memory usage in Java applications.
Module 7: Java Networking
- Introduction to Networking in Java
- Understanding IP addresses, sockets, and ports.
- Java’s networking API:
java.net
package.
- TCP and UDP Communication
- Client-server model using TCP sockets.
- Datagram sockets (UDP) and their use cases.
- Java Web Applications
- Introduction to Java web technologies: Servlets and JSP.
- Creating basic client-server applications using Java.
Module 8: Java Database Connectivity (JDBC)
- Introduction to JDBC
- Understanding the JDBC architecture.
- Setting up a connection to the database.
- Basic JDBC Operations
- Executing SQL queries: SELECT, INSERT, UPDATE, DELETE.
- Handling result sets.
- PreparedStatements and Statement objects.
- Transaction Management
- Managing transactions in JDBC.
- Committing and rolling back transactions.
- JDBC with Connection Pooling
- Using connection pools for optimized database connections.
Module 9: Java Frameworks and Libraries
Spring Framework
- Overview of Spring: Inversion of Control (IoC) and Dependency Injection (DI).
- Creating simple Spring-based applications.
Hibernate ORM
- Introduction to Object-Relational Mapping (ORM).
- Mapping Java objects to database tables.
- Hibernate configuration and entity management.
Apache Maven and Gradle
- Using Maven for project dependency management.
- Creating and managing build scripts with Gradle.
JUnit Testing Framework
- Writing unit tests in Java using JUnit.
- Mocking dependencies with Mockito.
Module 10: Java Web Development
Introduction to Web Development with Java
- Understanding the role of Servlets and JSP in web applications.
- Handling HTTP requests and responses.
RESTful Web Services with Spring Boot
- Building REST APIs using Spring Boot.
- Using
@RestController
,@RequestMapping
, and@PathVariable
.
Web Security with Spring Security
- Authentication and authorization in web applications.
- Securing REST APIs with JWT and OAuth2.
Deploying Java Web Applications
- Deploying Java applications to web servers like Tomcat.
- Building and deploying a Spring Boot application.
Module 11: Best Practices and Java Design Patterns
Java Coding Best Practices
- Writing clean, readable, and efficient code.
- Following naming conventions and style guidelines.
Design Patterns in Java
- Overview of design patterns and their importance.
- Common design patterns: Singleton, Factory, Observer, Strategy, Decorator.
SOLID Principles
- Explanation of SOLID principles for object-oriented design.
- Applying SOLID principles in Java applications.
Module 12: Java Performance Optimization
Performance Tuning
- Identifying performance bottlenecks.
- Profiling Java applications using tools like VisualVM and JProfiler.
Java Optimization Techniques
- Memory and CPU optimization in Java applications.
- Optimizing I/O performance and network communication.
Concurrency and Parallel Processing
- Parallel programming using Java Streams API.
- Efficient thread usage and minimizing synchronization overhead.
40 Days (also available fast track course with short term duration)
- Flexible Schedules
- Live Online Training
- Training by highly experienced and certified professionals
- No slideshow (PPT) training, fully Hand-on training
- Interactive session with interview QA’s
- Real-time projects scenarios & Certification Help
- 24 X 7 Support