Microsoft .Net
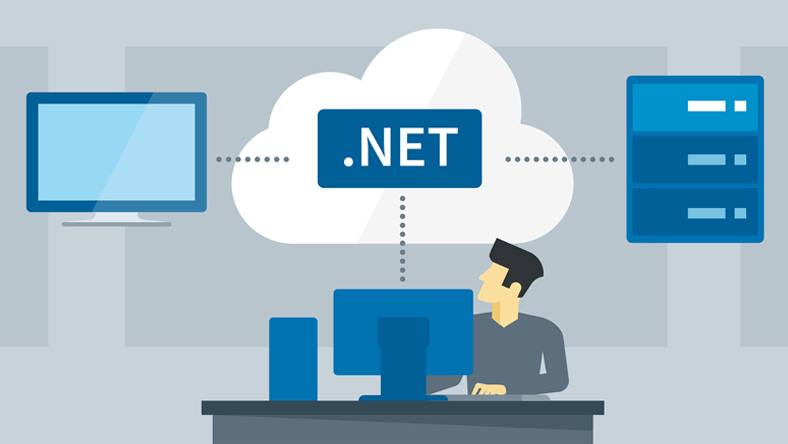
Contact Form
Microsoft .NET is a versatile framework that provides a comprehensive set of tools for building and running applications across various platforms. It supports multiple programming languages and offers a robust runtime environment, libraries, and APIs. .NET applications run on Windows, Linux, and macOS, with integrated security features and extensive support from Microsoft, ensuring cross-platform compatibility and reliability.
- Basic knowledge of any programming language is beneficial.
- A fundamental understanding of computer science principles, such as algorithms and data structures, as well as basic concepts of networking and software development, will also be helpful for learning Microsoft .NET.
Module 1: Introduction to .NET
Overview of .NET
- What is .NET and its evolution (from .NET Framework to .NET Core to .NET 5/6/7).
- Features of .NET: Cross-platform, Open-source, High performance.
- Understanding the .NET Ecosystem: .NET SDK, CLI, NuGet, and runtime.
Setting Up Development Environment
- Installing Visual Studio (Community/Enterprise).
- Setting up the .NET SDK and working with the command-line interface (CLI).
- Overview of Visual Studio tools for .NET development.
Module 2: .NET Core Fundamentals
Basic .NET Core Concepts
- What is .NET Core? Benefits and differences from the .NET Framework.
- Architecture: CLR (Common Language Runtime), BCL (Base Class Library), and assembly.
- Understanding the role of the .NET runtime and just-in-time (JIT) compilation.
Creating a Simple .NET Core Application
- Creating a “Hello World” console application.
- Understanding project structure: .csproj, Program.cs, Startup.cs.
- Building and running a console application.
Basic Syntax and C# Basics
- Introduction to C#: Variables, Data types, Constants, and Operators.
- Control structures:
if
,switch
, loops (for
,while
,foreach
). - Methods, functions, and parameters.
- Exception handling (
try
,catch
,finally
).
Module 3: Object-Oriented Programming (OOP) in .NET
Understanding OOP Principles
- Encapsulation, Abstraction, Inheritance, and Polymorphism in .NET.
- Creating and using classes and objects.
- Constructors and destructors in C#.
Inheritance and Interfaces
- Creating subclasses and overriding methods.
- Implementing interfaces and abstract classes.
Polymorphism
- Method overloading and method overriding.
- Understanding static vs dynamic polymorphism.
Delegates and Events
- Introduction to delegates and events in C#.
- Defining and using delegates.
- Event handling in C#.
Module 4: Advanced C# Features
Generics
- Introduction to generics: Benefits and use cases.
- Generic classes, methods, and interfaces.
- Constraints in generics.
LINQ (Language Integrated Query)
- Introduction to LINQ and its capabilities.
- Querying collections: Arrays, Lists, Dictionaries, etc.
- LINQ with method syntax and query syntax.
Collections in .NET
- Understanding collections: Arrays, Lists, Dictionaries, HashSets, Queues, and Stacks.
- Working with generics in collections.
Working with Dates and Time
- DateTime structure and its methods.
- TimeSpan and manipulating time intervals.
Module 5: .NET Core Web Development
Introduction to ASP.NET Core
- Understanding the ASP.NET Core framework.
- The role of MVC (Model-View-Controller) in web applications.
- Setting up an ASP.NET Core Web API project.
Creating RESTful APIs with ASP.NET Core
- Building APIs using controllers and routing.
- HTTP methods (GET, POST, PUT, DELETE) in RESTful services.
- Handling JSON requests and responses.
- Data validation and error handling in APIs.
Middleware in ASP.NET Core
- What is middleware and how it works in the request pipeline?
- Creating custom middleware.
- Using built-in middleware components like logging, authentication, and routing.
Dependency Injection (DI)
- What is Dependency Injection and why is it important in ASP.NET Core?
- Configuring services in
Startup.cs
for DI. - Using constructor injection, property injection, and method injection.
Module 6: Entity Framework Core
- Introduction to Entity Framework Core
- What is ORM (Object-Relational Mapping) and the role of EF Core.
- Setting up EF Core with a SQL database.
- Working with Databases in EF Core
- Creating models and DbContext.
- Using migrations to create and update database schemas.
- Performing CRUD operations with EF Core.
- Querying with LINQ and EF Core
- Writing LINQ queries for EF Core.
- Filtering, sorting, and pagination in queries.
- Advanced EF Core Features
- Handling relationships (One-to-One, One-to-Many, Many-to-Many).
- Eager loading vs Lazy loading.
- Tracking and non-tracking queries.
Module 7: Unit Testing and Test-Driven Development (TDD)
Introduction to Unit Testing
- What is unit testing and why is it important in software development?
- Writing unit tests using xUnit or NUnit.
Test-Driven Development (TDD)
- The TDD process: Write a failing test, write code to pass the test, and refactor.
- Creating unit tests for business logic and controllers.
- Mocking dependencies using libraries like Moq.
Integration Testing
- Setting up integration tests for ASP.NET Core APIs.
- Testing database interactions with EF Core.
Module 8: Asynchronous Programming in .NET
Async and Await
- Understanding the asynchronous programming model.
- Using the
async
keyword andawait
for non-blocking code.
Task-based Asynchronous Pattern (TAP)
- Working with
Task
andTask<T>
for asynchronous operations. - Handling multiple asynchronous operations with
Task.WhenAll()
andTask.WhenAny()
.
- Working with
Handling Deadlocks and Exceptions in Async Programming
- Avoiding common pitfalls and deadlocks.
- Handling exceptions in async code with
try
,catch
,finally
.
Module 9: Security in .NET Applications
Authentication and Authorization
- Authentication in ASP.NET Core using Identity.
- Implementing role-based and policy-based authorization.
- Using JWT (JSON Web Tokens) for secure authentication.
Securing APIs with OAuth2 and OpenID Connect
- Implementing OAuth2 and OpenID Connect in web applications.
- Using IdentityServer4 for OAuth and OpenID Connect in .NET.
Data Encryption and Hashing
- Understanding encryption algorithms: AES, RSA.
- Using
System.Security.Cryptography
for encryption and hashing.
Module 10: .NET Core MVC and Web Development
Understanding MVC Architecture
- Building Model-View-Controller (MVC) applications.
- Views and Razor Pages in ASP.NET Core.
- Routing and URL patterns.
Working with Views
- Creating views with Razor syntax.
- Partial views and layout pages.
Handling Forms and Validation
- Creating forms in Razor views.
- Model binding and validation attributes.
Module 11: Deploying .NET Core Applications
Deploying Web Applications to Azure
- Setting up an Azure account.
- Deploying a .NET Core application to Azure App Service.
Containerization with Docker
- What is Docker? Introduction to containers.
- Creating Docker images for .NET Core applications.
- Running .NET Core applications in Docker containers.
CI/CD with Azure DevOps
- Setting up Continuous Integration and Continuous Deployment pipelines with Azure DevOps.
- Automating testing and deployment of .NET Core applications.
40 Days (also available fast track course with short term duration)
- Flexible Schedules
- Live Online Training
- Training by highly experienced and certified professionals
- No slideshow (PPT) training, fully Hand-on training
- Interactive session with interview QA’s
- Real-time projects scenarios & Certification Help
- 24 X 7 Support